Quickstart
This page will guide you through the process of getting started with TilemapExtended. Have a look at the tutorial if you prefer a more in-depth guide.
Define
After importing the asset package, define a custom data class to hold the data you need per Tile:
- Create a new C# script and extend the
CellData
ScriptableObject. - Add all your custom properties and methods.
- Create an asset menu in order to create object instances in the editor.
An example could look like this:
using TExtended;
using UnityEngine;
[CreateAssetMenu(menuName = "CellData/MyCellData", fileName = "New MyCellData")]
public class MyCellData : CellData {
public TerrainType terrainType;
public bool canBuildOn;
public int fertility;
}
Create object instances in the editor and modify values according to your needs, for example different kinds of Tiles: Grass, Sand, Water, Rock, etc.
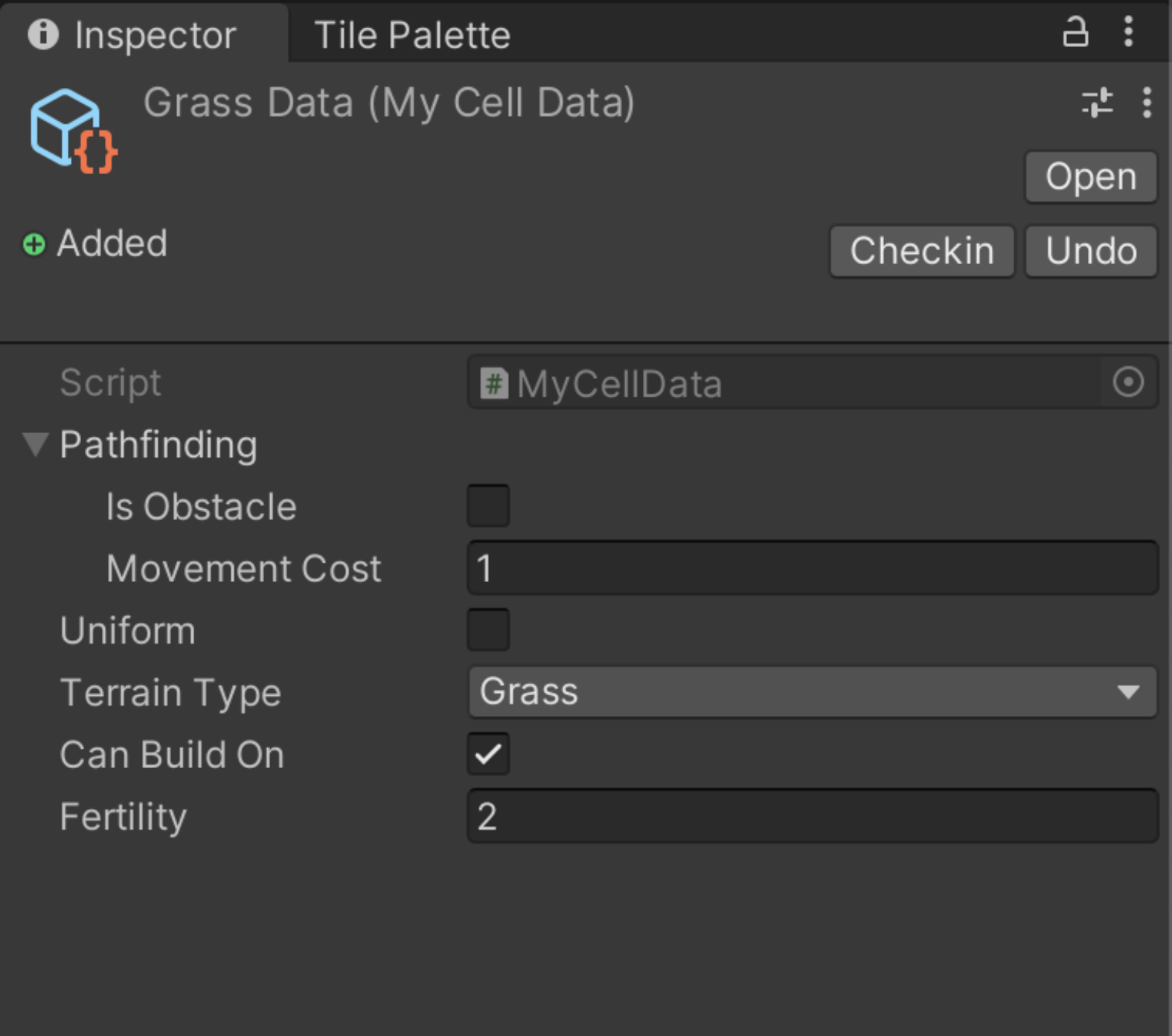
Assign
To link your newly created data objects to any Tiles, create rules within a pre-made container object: Create > TilemapExtended > TilemapRulesContainer.
Add elements (rules) within this container and drag the data object into the CellData
field. Add elements to the
Tiles
field and drag any Unity Tiles you wish to link to the data object.
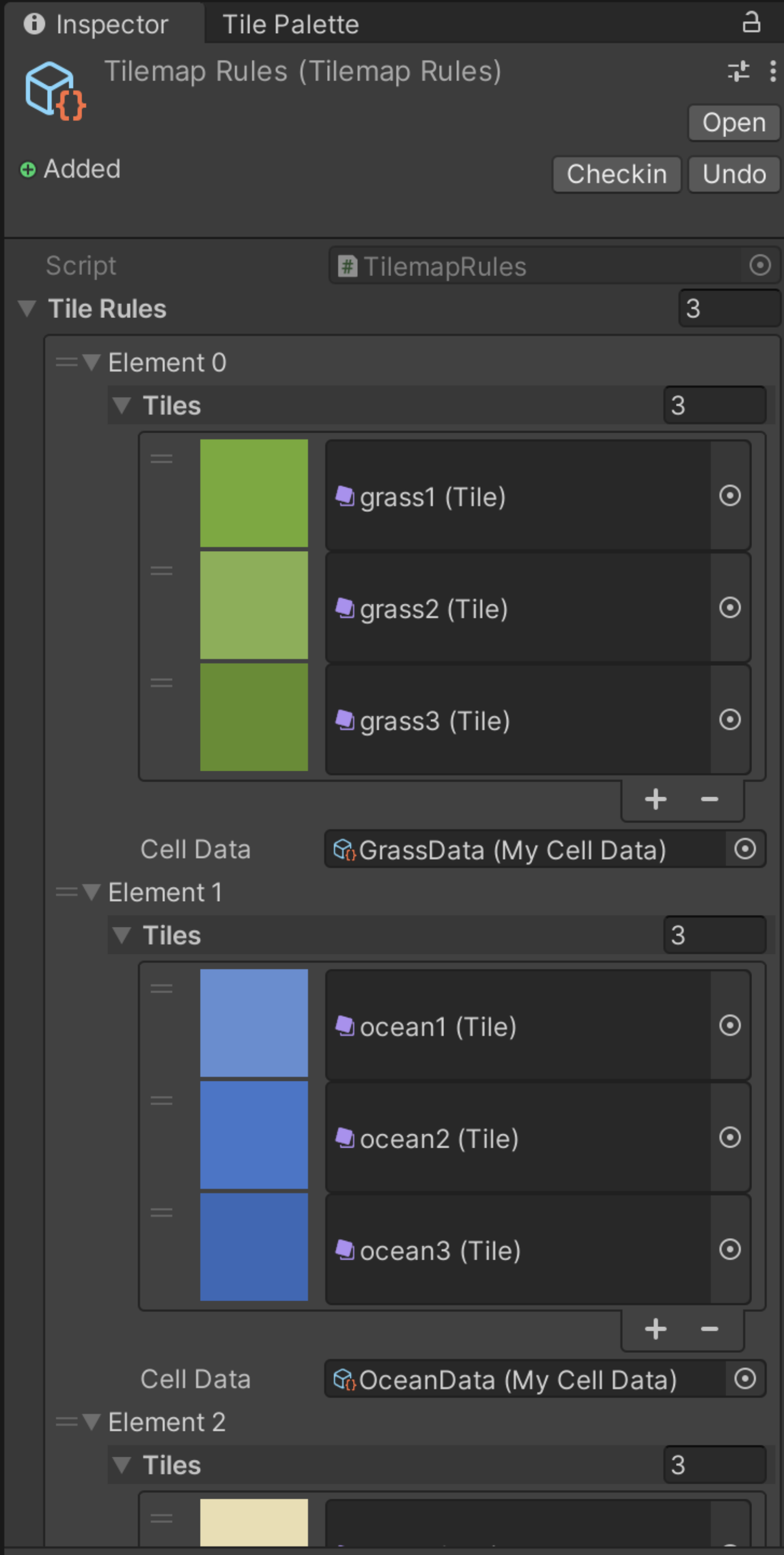
Drag the TilemapExtended prefab containing the controller script into the scene hierarchy and drag your rule container
into the TileRules
field. If your Unity Grid component is named "Grid", simply hit Detect
and the Grid and all
enabled Tilemaps will be added automatically. Alternatively, add the components manually. The layer sorting order is not
important and will be handled internally at runtime.
Use
In your controller script, create a reference to the TilemapExtended
script component of the TilemapExtended game
object. After entering play mode, the Tilemaps will be scanned and you get full access to your data cells by
calling TilemapExtended.DataCells. Call TilemapExtended.GridExtended for useful grid methods and utilities.
Data cells are stored as their CellData
base class. In order to access your data, cast them to your custom class:
var gridPosition = new Vector3Int(1, 4, 0);
var cell = TilemapExtended.DataCells.GetCell(gridPosition) as MyCellData;
Here's an example in which neighbour cells are retrieved and modified:
TilemapExtended = GameObject.Find("TilemapExtended").GetComponent<TilemapExtended>();
var gridPosition = new Vector3Int(1, 2, 0);
//Returns a list of neighbouring cells
var neighbours = TilemapExtended.GridExtended.GetNeighbours(gridPosition);
//Iterate neighbour cells to check for TerrainType
foreach (var neighbour in neighbours) {
var neighbourData = neighbour as MyCellData;
if (neighbourData.terrainType == TerrainType.Ocean) {
neighbourData.fertility += 1;
}
}
This short introduction is meant to get you started with this tools, please see the tutorial for a more in-depth guide or have a look at the API documentation.